指定したフォルダー配下にあるファイル数をリアルタイムで監視するツールを作成したので公開します。
ツールについて
本ツールについて簡単に説明します。
まず、以下がツールの実行イメージです。
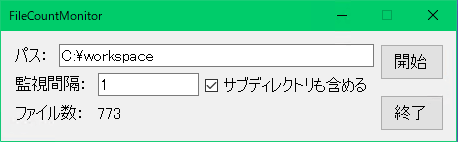
使い方は、監視したいフォルダーパスと監視間隔(秒)を入力し、開始ボタンをクリックするだけです。
オプションとして、サブディレクトリ配下も監視対象にするかを選択するチェックボックスも付けました。
ソースコード
以下が、本ツールのソースコードです。
.NET Framework 4.8 環境で実装しました。
using System;
using System.IO;
using System.Windows.Forms;
using System.Timers;
namespace Form1
{
public partial class Form1 : Form
{
private string selectedFolder;
private readonly System.Timers.Timer timer;
private bool isRecursive;
public Form1()
{
InitializeComponent();
timer = new System.Timers.Timer();
timer.Elapsed += OnTimedEvent;
chkRecursive.CheckedChanged += chkRecursive_CheckedChanged;
}
private void btnStart_Click(object sender, EventArgs e)
{
// テキストボックスからフォルダパスを取得
selectedFolder = txtFolderPath.Text;
// パスが存在しない場合の処理
if (!Directory.Exists(selectedFolder))
{
MessageBox.Show("指定したフォルダが存在しません。");
return;
}
// 監視間隔の取得
if (int.TryParse(txtInterval.Text, out int interval))
{
// 秒単位をミリ秒に変換
timer.Interval = interval * 1000;
// 監視開始
timer.Start();
}
else
{
MessageBox.Show("監視間隔を正しい数値で入力してください。");
}
}
private void btnStop_Click(object sender, EventArgs e)
{
// 監視停止
timer.Stop();
lblResult.Text = "待機中";
}
private void OnTimedEvent(object sender, ElapsedEventArgs e)
{
// ファイル数の取得
int fileCount = isRecursive
? Directory.GetFiles(selectedFolder, "*", SearchOption.AllDirectories).Length
: Directory.GetFiles(selectedFolder).Length;
// ファイル数の表示
Invoke(new Action(() => lblResult.Text = fileCount.ToString()));
}
private void chkRecursive_CheckedChanged(object sender, EventArgs e)
{
// サブディレクトリを監視対象に含めるか
isRecursive = chkRecursive.Checked;
}
}
}
ソースコード(デザイン)
こちらは、フォームアプリのデザインコードです。
namespace Form1
{
partial class Form1
{
/// <summary>
/// 必要なデザイナー変数です。
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// 使用中のリソースをすべてクリーンアップします。
/// </summary>
/// <param name="disposing">マネージド リソースを破棄する場合は true を指定し、その他の場合は false を指定します。</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows フォーム デザイナーで生成されたコード
/// <summary>
/// デザイナー サポートに必要なメソッドです。このメソッドの内容を
/// コード エディターで変更しないでください。
/// </summary>
private void InitializeComponent()
{
this.btnStart = new System.Windows.Forms.Button();
this.btnStop = new System.Windows.Forms.Button();
this.txtInterval = new System.Windows.Forms.TextBox();
this.chkRecursive = new System.Windows.Forms.CheckBox();
this.lblResult = new System.Windows.Forms.Label();
this.txtFolderPath = new System.Windows.Forms.TextBox();
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// btnStart
//
this.btnStart.Font = new System.Drawing.Font("MS UI Gothic", 12F);
this.btnStart.Location = new System.Drawing.Point(379, 13);
this.btnStart.Name = "btnStart";
this.btnStart.Size = new System.Drawing.Size(64, 36);
this.btnStart.TabIndex = 1;
this.btnStart.Text = "開始";
this.btnStart.UseVisualStyleBackColor = true;
this.btnStart.Click += new System.EventHandler(this.btnStart_Click);
//
// btnStop
//
this.btnStop.Font = new System.Drawing.Font("MS UI Gothic", 12F);
this.btnStop.Location = new System.Drawing.Point(379, 64);
this.btnStop.Name = "btnStop";
this.btnStop.Size = new System.Drawing.Size(64, 36);
this.btnStop.TabIndex = 2;
this.btnStop.Text = "終了";
this.btnStop.UseVisualStyleBackColor = true;
this.btnStop.Click += new System.EventHandler(this.btnStop_Click);
//
// txtInterval
//
this.txtInterval.Font = new System.Drawing.Font("MS UI Gothic", 12F);
this.txtInterval.Location = new System.Drawing.Point(97, 42);
this.txtInterval.Name = "txtInterval";
this.txtInterval.Size = new System.Drawing.Size(101, 23);
this.txtInterval.TabIndex = 3;
//
// chkRecursive
//
this.chkRecursive.AutoSize = true;
this.chkRecursive.Font = new System.Drawing.Font("MS UI Gothic", 12F);
this.chkRecursive.Location = new System.Drawing.Point(204, 45);
this.chkRecursive.Name = "chkRecursive";
this.chkRecursive.Size = new System.Drawing.Size(169, 20);
this.chkRecursive.TabIndex = 4;
this.chkRecursive.Text = "サブディレクトリも含める";
this.chkRecursive.UseVisualStyleBackColor = true;
//
// lblResult
//
this.lblResult.AutoSize = true;
this.lblResult.Font = new System.Drawing.Font("MS UI Gothic", 12F);
this.lblResult.Location = new System.Drawing.Point(94, 74);
this.lblResult.Name = "lblResult";
this.lblResult.Size = new System.Drawing.Size(55, 16);
this.lblResult.TabIndex = 5;
this.lblResult.Text = "待機中";
//
// txtFolderPath
//
this.txtFolderPath.Font = new System.Drawing.Font("MS UI Gothic", 12F);
this.txtFolderPath.Location = new System.Drawing.Point(58, 13);
this.txtFolderPath.Name = "txtFolderPath";
this.txtFolderPath.Size = new System.Drawing.Size(315, 23);
this.txtFolderPath.TabIndex = 6;
//
// label1
//
this.label1.AutoSize = true;
this.label1.Font = new System.Drawing.Font("MS UI Gothic", 12F);
this.label1.Location = new System.Drawing.Point(12, 16);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(40, 16);
this.label1.TabIndex = 7;
this.label1.Text = "パス:";
//
// label2
//
this.label2.AutoSize = true;
this.label2.Font = new System.Drawing.Font("MS UI Gothic", 12F);
this.label2.Location = new System.Drawing.Point(12, 45);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(79, 16);
this.label2.TabIndex = 8;
this.label2.Text = "監視間隔:";
//
// label3
//
this.label3.AutoSize = true;
this.label3.Font = new System.Drawing.Font("MS UI Gothic", 12F);
this.label3.Location = new System.Drawing.Point(12, 74);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(76, 16);
this.label3.TabIndex = 9;
this.label3.Text = "ファイル数:";
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 12F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(455, 110);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Controls.Add(this.txtFolderPath);
this.Controls.Add(this.lblResult);
this.Controls.Add(this.chkRecursive);
this.Controls.Add(this.txtInterval);
this.Controls.Add(this.btnStop);
this.Controls.Add(this.btnStart);
this.FormBorderStyle = System.Windows.Forms.FormBorderStyle.FixedDialog;
this.MaximizeBox = false;
this.Name = "Form1";
this.Text = "FileCountMonitor";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Button btnStart;
private System.Windows.Forms.Button btnStop;
private System.Windows.Forms.TextBox txtInterval;
private System.Windows.Forms.CheckBox chkRecursive;
private System.Windows.Forms.Label lblResult;
private System.Windows.Forms.TextBox txtFolderPath;
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
}
}
コメント