C#で、CSVファイルの読み込みと書き込みする方法を解説します。
CSVの読み込み
ここでは、CSVを読み込む方法を説明します。
事前準備
事前準備として、ボタンとリッチテキストボックスを配置した簡単な画面を作成します。
ボタンは処理の開始用、リッチテキストボックスは読み込んだ内容の表示用です。
以下は、デザインのコードです。
namespace WinFormsApp2
{
partial class Form1
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
richTextBox1 = new RichTextBox();
button1 = new Button();
SuspendLayout();
//
// richTextBox1
//
richTextBox1.Font = new Font("Yu Gothic UI", 14.25F, FontStyle.Regular, GraphicsUnit.Point, 128);
richTextBox1.Location = new Point(12, 12);
richTextBox1.Name = "richTextBox1";
richTextBox1.Size = new Size(342, 207);
richTextBox1.TabIndex = 0;
richTextBox1.Text = "";
//
// button1
//
button1.Location = new Point(220, 12);
button1.Name = "button1";
button1.Size = new Size(134, 105);
button1.TabIndex = 1;
button1.Text = "button1";
button1.UseVisualStyleBackColor = true;
button1.Click += button1_Click;
//
// Form1
//
AutoScaleDimensions = new SizeF(7F, 15F);
AutoScaleMode = AutoScaleMode.Font;
ClientSize = new Size(366, 231);
Controls.Add(button1);
Controls.Add(richTextBox1);
Name = "Form1";
Text = "Form1";
ResumeLayout(false);
}
#endregion
private RichTextBox richTextBox1;
private Button button1;
}
}
ソースコード
読み込んだCSVファイルの内容をリッチテキストボックスに表示するソースコードです。
using System.Diagnostics;
namespace WinFormsApp2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// CSVファイルを読み込む
ReadCsv();
}
// CSVファイルを読み込む
private void ReadCsv()
{
// 読み込むCSVファイル
string csv = @"C:\Workspace\input.csv";
// インスタンスの作成
using StreamReader reader = new(csv);
// CSVを読み込み1行ずつ最終行までループ
while (!reader.EndOfStream)
{
// 読み込んだCSVの行情報
string line = reader.ReadLine() ?? "";
// 行をカンマ区切りで分割
string[] values = line.Split(',');
// CSVのカラムごとにループ
foreach (string value in values)
{
// リッチテキストボックスに出力
richTextBox1.AppendText(value + " ");
}
// リッチテキストボックスに改行を出力
richTextBox1.AppendText("\n");
}
}
}
}
実行イメージ
ボタンを押し、CSVが読み込まれた時の実行イメージです。
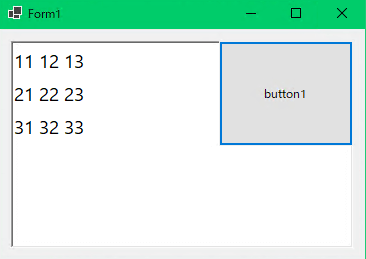
CSVの書き込み
ここでは、CSVを書き出す方法を説明します。
事前準備
事前準備として、処理を実行するためのボタンを配置した簡単な画面を作成します。
以下は、デザインのコードです。
namespace WinFormsApp2
{
partial class Form1
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
button1 = new Button();
SuspendLayout();
//
// button1
//
button1.Location = new Point(12, 12);
button1.Name = "button1";
button1.Size = new Size(147, 101);
button1.TabIndex = 0;
button1.Text = "button1";
button1.UseVisualStyleBackColor = true;
button1.Click += button1_Click;
//
// Form1
//
AutoScaleDimensions = new SizeF(7F, 15F);
AutoScaleMode = AutoScaleMode.Font;
ClientSize = new Size(325, 228);
Controls.Add(button1);
Name = "Form1";
Text = "Form1";
ResumeLayout(false);
}
#endregion
private Button button1;
}
}
ソースコード
ボタンを押すと、CSVファイルを出力するソースコードです。
namespace WinFormsApp2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// CSVの書き出し
WriteCSV();
}
// CSVの書き出し
private void WriteCSV()
{
// 書き出すCSVファイル
string csv = @"C:\Workspace\output.csv";
// インスタンスの作成
using StreamWriter writer = new(csv);
// CSVファイルへの書き込み
writer.WriteLine("11,12,13");
writer.WriteLine("21,22,23");
writer.WriteLine("31,32,33");
}
}
}
実行イメージ
出力したCSVファイルのイメージです。
11,12,13 21,22,23 31,32,33
リンク
コメント